Kotlin의 클래스와 생성자에 대하여 알아보아요.
Kotlin 클래스와 생성자
클래스
class
키워드
- Kotlin 클래스는
class
키워드를 사용하여 선언합니다.
- 클래스 선언부는 아래와 같이 구성됩니다.
- 클래스 이름
- 클래스 헤더(클래스 타입 파라미터
<T>
, 기본 생성자 constructor
등),
- 중괄호
{ }
로 둘러 싸인 클래스 바디
- 클래스 헤더와 바디는 optional이므로, 바디가 없다면 중괄호
{ }
를 생략할 수 있습니다.
Kotlin 클래스 생성하기
- Intellij 상단 메뉴에서
[File] - [New] - [Kotlin File/Class]
클릭합니다.
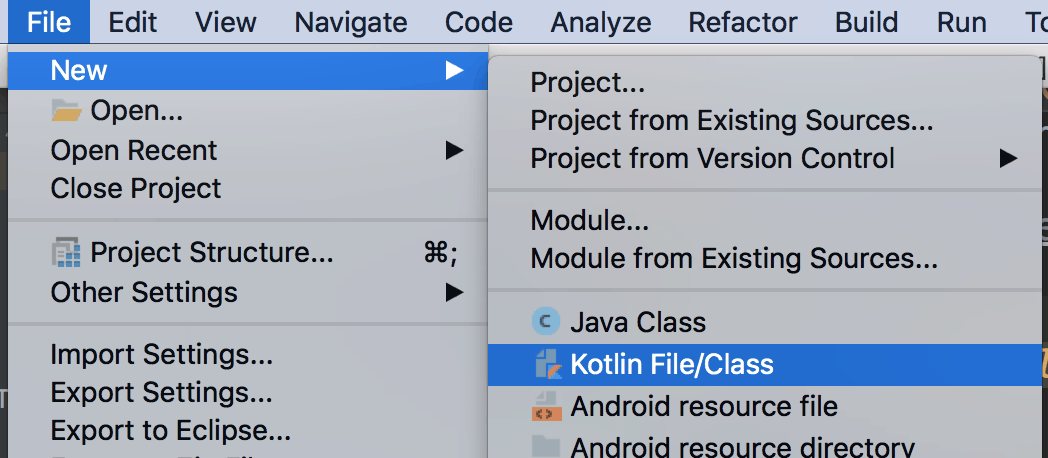
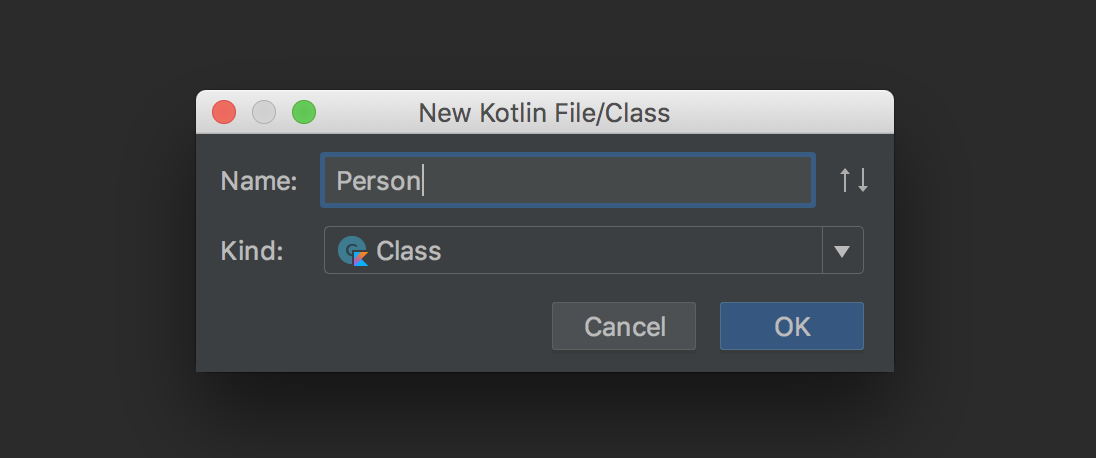
1
2
3
4
| // Person.kt
class Person {
}
|
생성자
constructor
키워드
- Java와 비교 - Java는 생성자를 클래스 내부에 선언해야합니다.
1
2
3
4
5
6
| // Person.java
public class Person {
Person(String firstName) {
...
}
}
|
- Kotlin은
constructor
키워드를 사용하여 생성자를 클래스 선언부(기본 생성자)나 클래스 내부(보조 생성자)에 선언할 수 있습니다.
1
2
3
4
| // Person.kt - 기본 생성자
class Person constructor(firstName: String) {
...
}
|
기본 생성자(primary constructor)
- 기본 생성자는 클래스 헤더의 한 부분으로, 클래스 이름 뒤(타입 파라미터
<T>
가 있다면 그 뒤)에 위치합니다.
1
| class Person constructor(firstName: String) { ... }
|
- 기본 생성자에 애노테이션(annotations)이나 접근 제한자(visibility modifiers)가 없다면
constructor
키워드를 생략할 수 있습니다.
1
2
3
4
| // Person.kt - constructor 키워드 생략 가능
class Person(firstName: String) {
...
}
|
1
2
3
4
| // Customer.kt - constructor 키워드 생략 불가능
class Customer public @Inject constructor(name: String) {
...
}
|
- 기본 생성자의 파라미터는 클래스 바디에 선언된 프로퍼티 초기화에도 사용할 수 있습니다.
1
2
3
4
| class Customer(name: String) {
// 프로퍼티 customerKey 초기화에 name 파리미터 사용
val customerKey = name.toUpperCase()
}
|
- 코틀린은 기본 생성자에서 프로퍼티를 선언하고 초기화하는 간결한 구문을 제공합니다.
(var
와 val
)
1
2
3
| class Person(val firstName: String, val lastName: String, var age: Int) {
...
}
|
init
키워드
- 코틀린에서는 기본 생성자가 클래스 선언부에 위치하므로 바디를 가질 수 없는 구조입니다.
- 대신,
init{}
함수 안에 초기화 코드를 작성하여 기본 생성자의 인자값을 처리할 수 있습니다.
- 인스턴스가 초기화되는 동안,
init{}
함수는 프로퍼티 초기화와 함께 클래스 바디에 선언된 순서대로 실행됩니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
| class InitOrderDemo(name: String) {
val firstProperty = "First property: $name".also(::println)
init {
println("First initializer block that prints ${name}")
}
val secondProperty = "Second property: ${name.length}".also(::println)
init {
println("Second initializer block that prints ${name.length}")
}
}
|
실행결과
1
2
3
4
| First property: hello
First initializer block that prints hello
Second property: 5
Second initializer block that prints 5
|
보조 생성자(Secondary Constructors)
- 클래스 바디 내부에 보조생성자를 생성할 수 있습니다.
1
2
3
4
5
| class Person {
constructor(parent: Person) {
parent.children.add(this)
}
}
|
- 클래스에 기본 생성자가 있으면, 각 보조 생성자는
this
키워드를 사용하여 직접 기본생성자에게, 또는 다른 보조 생성자를 통해 간접적으로 기본생성자에게 위임을 해야합니다.
this
키워드는 기본 생성자의 속성을 상속받아 처리되는 형식입니다.
1
2
3
4
5
| class Person(val name: String) {
constructor(name: String, parent: Person) : this(name) {
parent.children.add(this)
}
}
|